Table of Contents
Introduction
Debugging Python for data professionals is key, whether they’re analysts, engineers, or working in machine learning, they all face various challenges when managing and analyzing data. Among these challenges, one common obstacle is encountering errors or bugs. While these may seem daunting at first, mastering the skill of debugging is essential in resolving issues and ensuring the quality and accuracy of data processes. This article walks through the basics of debugging, defines key terms, and outlines how to identify and describe errors for data professionals.
Who Are Data Professionals?
The term data professionals encompasses a broad group of people whose primary role involves working with data to generate insights or solve problems. This includes, but isn’t limited to, data analysts, engineers, data scientists, and statisticians. These individuals analyze, process, and interpret complex data sets to inform decision-making. As the field of data expands, so does the need for professionals who can efficiently troubleshoot errors, refine processes, and enhance the performance of data-driven systems.
Defining Key Terms
To understand debugging, it’s crucial to define the key concepts that are frequently encountered.
Debugging: Debugging is the methodical process of identifying, analyzing, and resolving bugs or errors in code, algorithms, or data pipelines. It involves understanding the root cause of the issue and finding ways to fix it, ensuring the data process functions as intended.
Error: An error is any issue or malfunction within a data process or code that prevents it from performing as expected. Errors may range from simple syntax mistakes in a script to more complex problems like misaligned data formats.
Bug: Often used interchangeably with error, a bug refers to a defect or flaw in a software system or script that leads to unexpected results. Bugs are essentially the reason why certain parts of a data process fail or produce incorrect outputs.
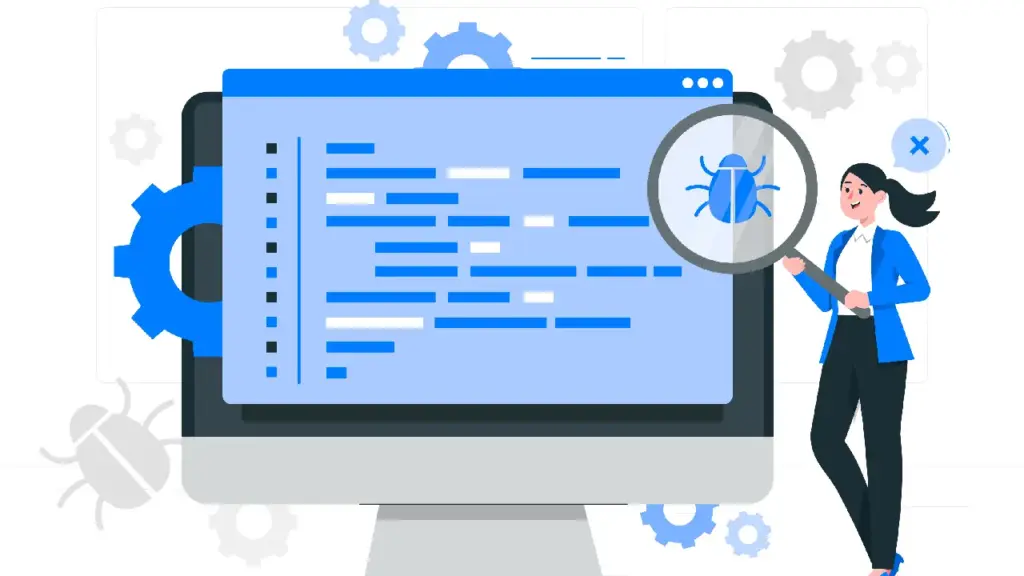
Common Python Errors and How to Fix Them
1. SyntaxError
A SyntaxError
occurs when there’s an issue with how your code is structured. Python is a language that’s sensitive to things like colons and indentation, and missing any of these can trigger this error.
Example:
for i in range(5)
print(i)
Notice the missing colon after the for
loop. Python expects certain symbols in specific places.
Fix: Add the colon so your loop is properly structured:
for i in range(5):
print(i)
2. NameError
You’ll see a NameError
when you try to use a variable or function that hasn’t been defined yet. This often happens because of a typo or because the variable wasn’t assigned properly.
Example:
print(total)
total = 50
In this case, total
was used before it was defined.
Fix: Ensure variables are assigned before you use them:
total = 50
print(total)
3. TypeError
A TypeError
happens when you try to perform an operation on the wrong data type. For instance, adding a string and an integer won’t work.
Example:
age = "25"
print(age + 5)
Here, you’re trying to add a string to a number, which Python can’t handle.
Fix: Convert the string to an integer using the int()
function:
age = "25"
print(int(age) + 5)
4. IndexError
If you try to access an index that doesn’t exist in a list or other data structure, you’ll get an IndexError
.
Example:
my_list = [1, 2, 3]
print(my_list[5])
This list only has three elements, so index 5 is out of range.
Fix: Always check the length of your list or use error handling to avoid this issue:
if len(my_list) > 5:
print(my_list[5])
5. ValueError
A ValueError
pops up when a function receives the right data type but an inappropriate value.
Example:
int("abc")
Here, “abc” can’t be converted to an integer.
Fix: Before converting, check if the string can be converted:
if "abc".isnumeric():
print(int("abc"))
6. KeyError
A KeyError
occurs when you try to access a dictionary key that doesn’t exist.
Example:
my_dict = {'a': 1, 'b': 2}
print(my_dict['c'])
The dictionary doesn’t have a key 'c'
.
Fix: Use the .get()
method, which will return None
instead of throwing an error if the key isn’t found:
print(my_dict.get('c'))
7. AttributeError
If you call a method or access an attribute that doesn’t exist for a particular object, you’ll get an AttributeError
.
Example:
my_list = [1, 2, 3]
my_list.add(4) # 'list' objects don't have an 'add' method
Fix: Use the correct method, like append()
for lists:
my_list.append(4)
8. MemoryError
A MemoryError
happens when your program consumes more memory than your system can handle. This is especially common in data work with very large datasets.
Example:
large_list = [0] * (10**10)
Fix: Break down the task into smaller chunks or use more memory-efficient libraries like NumPy or Pandas:
import numpy as np
large_array = np.zeros(10**10)
9. ImportError / ModuleNotFoundError
These errors occur when Python can’t find the module you’re trying to import.
Example:
import pandas
If pandas isn’t installed, you’ll get an error.
Fix: Install the required module using pip:
pip install pandas
10. OverflowError
An OverflowError
arises when a calculation exceeds the limits of Python’s numerical capabilities.
Example:
import math
math.exp(1000)
Fix: Use libraries that can handle large numbers, such as NumPy, or scale your calculations:
import numpy as np
np.exp(1000)
Conclusion
Debugging is a vital skill for data professionals, ensuring that the data-driven solutions they create are accurate and reliable. Whether you’re a beginner or an experienced coder, understanding common Python errors and how to resolve them will improve your ability to work efficiently and prevent costly mistakes in data processing or analysis. As you gain more experience, debugging will become second nature, enabling you to focus more on insights and less on fixing code.
References
- Wright, J. (2020). Debugging Python for Data Science. O’Reilly Media.
- Barak, M., et al. (2019). Automating and Simplifying Debugging in Python. ACM Journal of Programming.